Custom JavaScript Examples
Given the scope of using JavaScript, we only provide a limited number of examples that can be used to modify your export output.
Through changes in the custom.js file you can make further theme adaptions that may otherwise not be easily achievable with only CSS. For example, you may want to integrate with other services (eg. Google Analytics). Alternatively, you may want to make css and JavaScript changes in combination to modify the output of the generated HTML export. If you’re interested in adding metadata to your export output, please see our related documentation.
This page outlines some examples which allow users to:
Add Link to Header Section
To add a link to the header section add the following code within the custom.js file
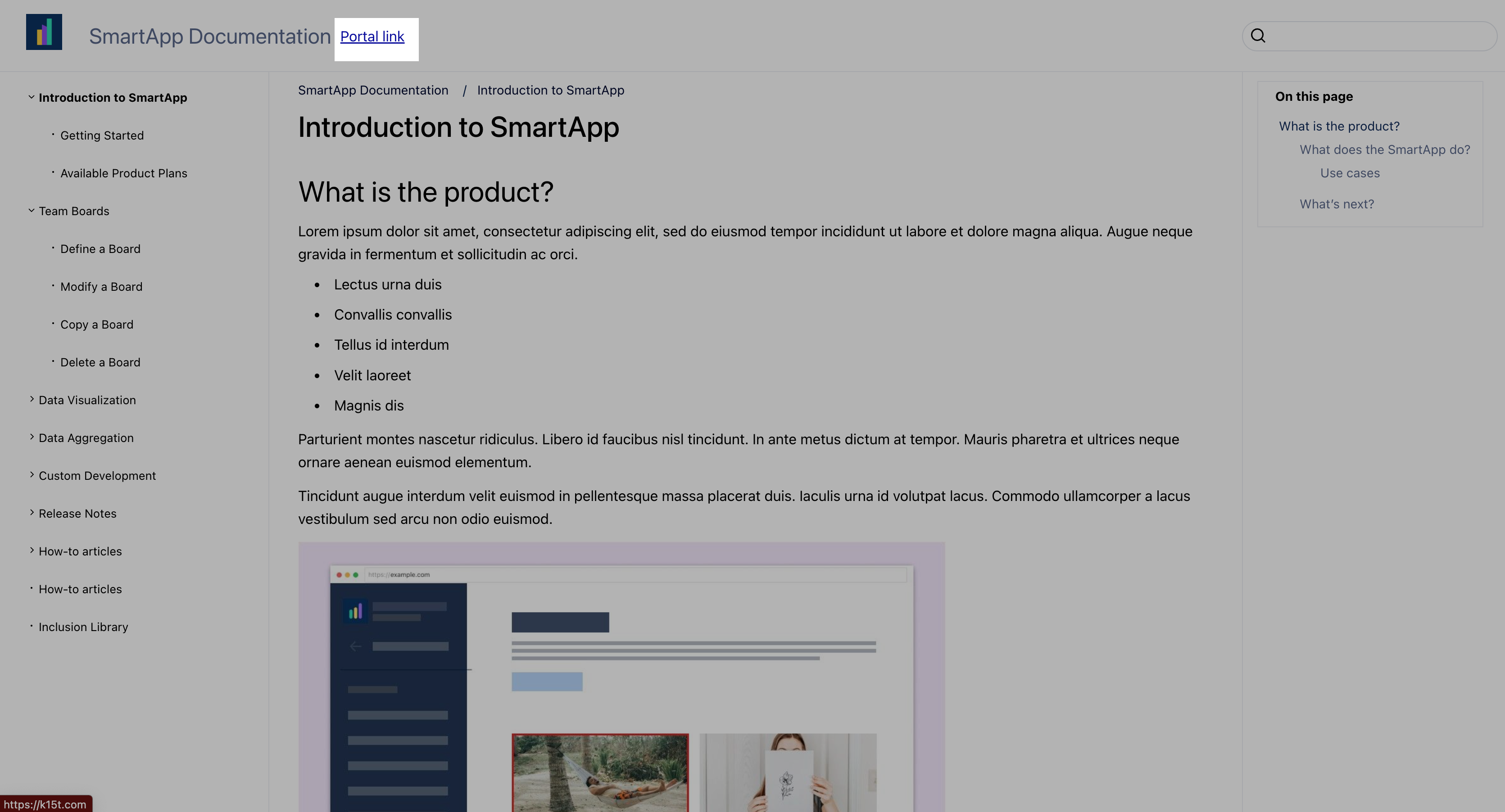
document.addEventListener('DOMContentLoaded', () => {
const LINK_TEXT = 'Portal link';
const LINK_URL = 'https://k15t.com';
const titleBar = document.querySelector('.exp-document-title');
if (titleBar) {
const link = document.createElement('a');
link.innerText = LINK_TEXT;
link.href = LINK_URL;
link.classList.add('header-link');
link.target = '_blank';
titleBar.appendChild(link);
}
}, false);
To format the added link add the following code within the custom.css file (learn more)
.exp-document-title {
display: flex;
flex-direction: row;
justify-content: flex-start;
align-items: center;
}
.header-link {
margin-left: 10px;
}
Remove Document Title from the Header Section
To remove the page title from the header section add the following code within the custom.js file
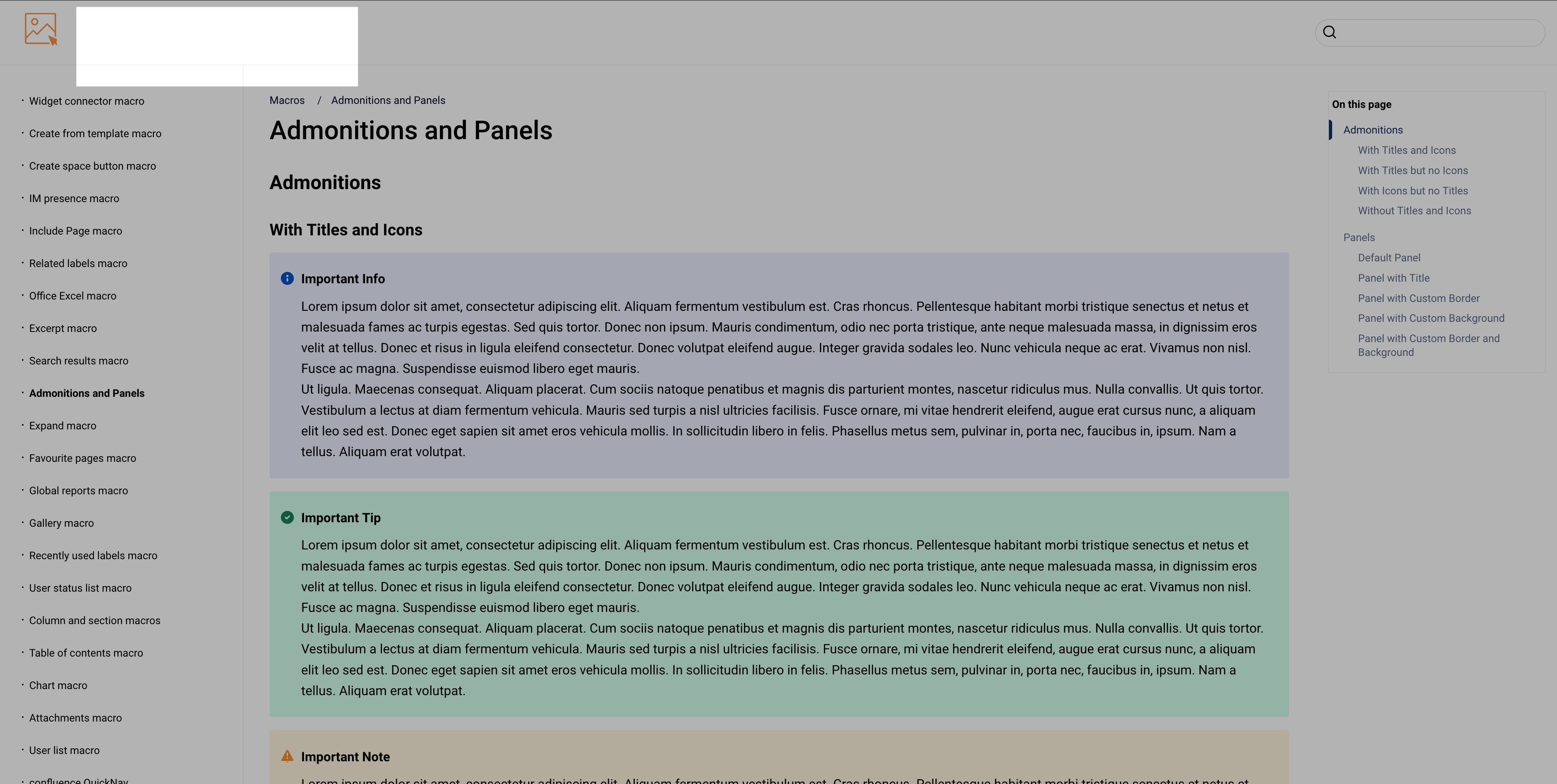
document.addEventListener('DOMContentLoaded', function() {
document.querySelector('.exp-document-title a').innerText = '';
});
Add Page Title to Header Section
To add heading text to the header section add the following code within the custom.js file
.png?inst-v=23bece65-240b-4281-b2b8-8aa791b6ad7e)
document.addEventListener('DOMContentLoaded', function() {
const spaceName = document.querySelector('meta[name="exp-page-title"]').content;
document.querySelector('.exp-document-title a').innerText = spaceName;
});
Add Static Text to Header Section
To add heading text to the header section, add the following code within the custom.js file
.png?inst-v=23bece65-240b-4281-b2b8-8aa791b6ad7e)
document.addEventListener('DOMContentLoaded', function() {
document.querySelector('.exp-document-title a').innerText = 'A custom header text';
});
Add Custom Image to Header
To add a custom image to the header, you can use two approaches.
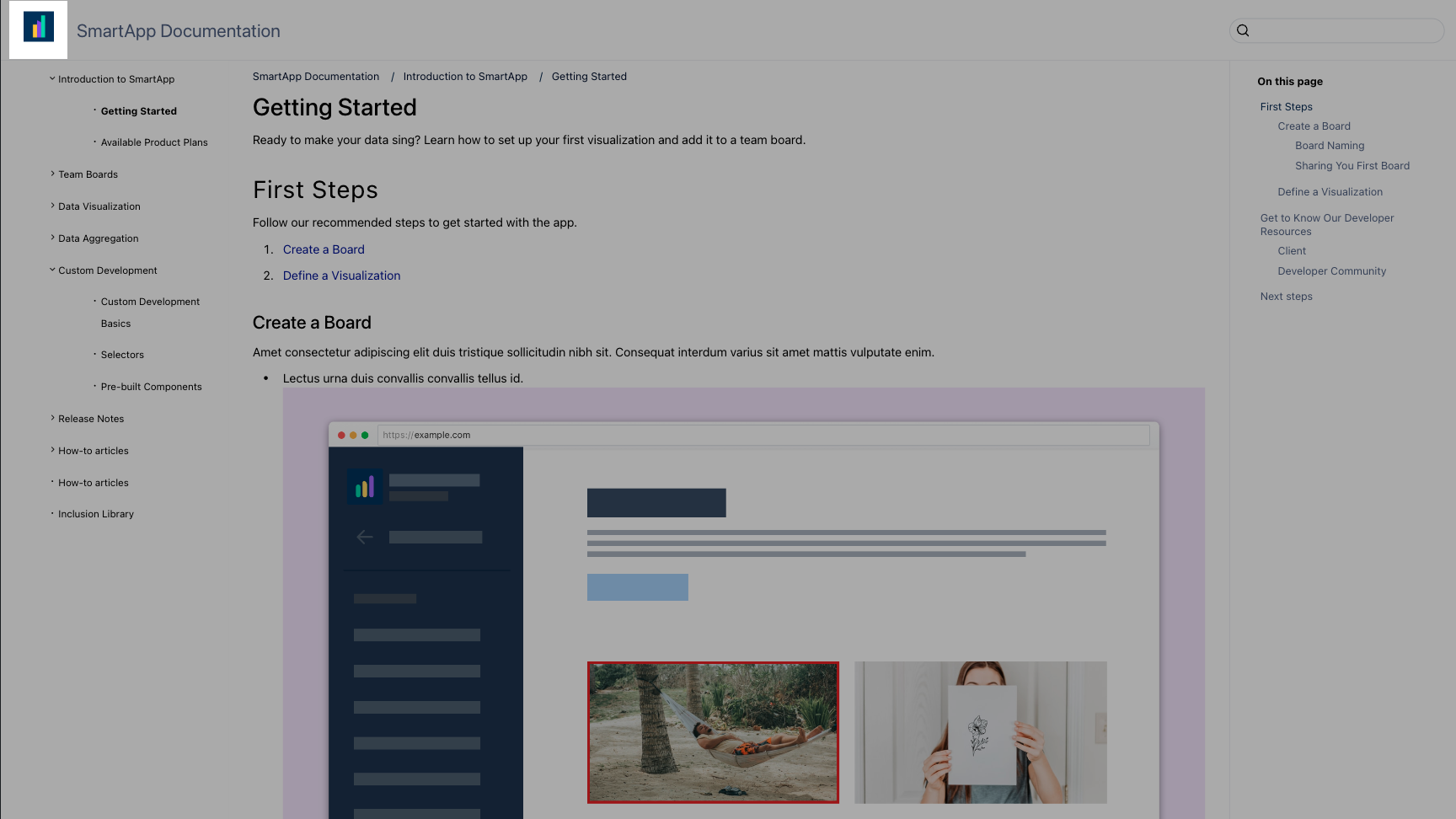
Include an image as a data URL:
Add the following code within the custom.js file:
document.addEventListener('DOMContentLoaded', function() {
document.querySelector(".exp-logo-image").style = "background-image: url(data:image/gif;base64,<GIF_IMAGE_AS_BASE64_HERE>)";
});
Include an image uploaded to the template:
Add the following code within the custom.js file:
document.addEventListener('DOMContentLoaded', function() {
document.querySelector(".exp-logo-image").style = "background-image: url(custom/images/uploaded-file-name.png)";
});