Exporting with Scroll Exporters from a Viewport
Using the code examples below, you can create a button to start exports from within your Viewport. For this recipe, we are going to use AUI dialogs as our UI – this depends on jQuery, but you are free to create or use any alternative.
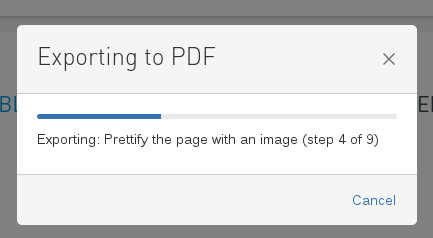
Export with Scroll PDF Exporter 4.x
Once you have created the export button, you can display the button in the viewport view.
Insert the required web resources
Firstly, insert the required web resources into the page.vm page of your template:
$page.resources.require("com.k15t.scroll.scroll-pdf:spark-export-ui-web-resource")
Include the export button
Now, you can to include the button code in the template wherever you want it to appear:
<button
class="aui-button scroll-pdf-launcher"
data-template-id="com.k15t.scroll.pdf.default-template-article"
data-space-key="$space.key"
data-page-id="$page.id"
data-scope="descendants"
data-quick-start="true"
>Export current page and children to PDF</button>
Defining the export settings
You can define various settings for the export:
Setting | Information |
---|---|
Template used | Insert the ID of the template you want to use in the To find the template ID, open Space Tools > Add-ons > Scroll PDF Exporter, find the template you want to use, open the actions dropdown menu, and click Template Information. |
Page exported | You can define a page to be exported by replacing |
Scope of export | Export either only defined page, or the defined page and its children. To export only the defined page, enter |
Quick Start | With the If the key is defined as " |
Export with other Scroll Exporters
Preparing the assets
Firstly, insert the webresources required for AUI in the head of your page.vm:
<head>
$page.resources.js
$page.resources.css
$page.resources.require('com.atlassian.auiplugin:dialog2')
$page.resources.require('com.atlassian.auiplugin:aui-progress-indicator')
</head>
Now, put the code for the dialog on the same page as your export button. You can also put it somewhere else to be later included as template.
<section role="dialog" id="demo-dialog" class="aui-layer aui-dialog2 aui-dialog2-small" aria-hidden="true">
<header class="aui-dialog2-header">
<h2 class="aui-dialog2-header-main">Export</h2>
<a class="aui-dialog2-header-close">
<span class="aui-icon aui-icon-small aui-iconfont-close-dialog">Close</span>
</a>
</header>
<div class="aui-dialog2-content">
<div id="progress-bar" class="aui-progress-indicator">
<span class="aui-progress-indicator-value"></span>
</div>
<p class="status-message"></p>
</div>
<footer class="aui-dialog2-footer">
<div class="aui-dialog2-footer-actions">
<button id="dialog-close-button" class="aui-button aui-button-link">Cancel</button>
</div>
</footer>
</section>
If your theme does not declare the window.contextPath
variable already, add this script tag in a .vm file. It must be evaluated before the JavaScript code from the next section.
<script>
window.contextPath = '$contextPath';
</script>
Implementing the code
The code below can be used in any <script>
tag or in an external javascript file. Browser compatibility has been tested in IE9+, Firefox, Chrome and Safari.
We use jQuery
instead of the global $
variable so you can paste this code into a script tag in any vm file. The $
variable causes issues in vm files because of the Velocity syntax. If you put this in a separate JavaScript file feel free to use $
instead.
jQuery(document).ready(function () {
if (jQuery('[data-exporter]').length) {
window.openExporter = function (link) {
var that = this;
this.link = link;
this.init = function () {
that.finished = false;
that.job = undefined;
that.link.preventDefault();
that.exporter = jQuery(that.link.target).data('exporter');
that.hash = that.link.target.href.substr(that.link.target.href.indexOf('#'));
that.pollInterval = 500;
that.restUrl = window.contextPath + '/rest';
that.restUrls = {
pdf: 'scroll-pdf/1.0',
office: 'scroll-office/1.0',
chm: 'scroll-chm/1.0',
html: 'scroll-html/1.0',
docbook: 'scroll-docbook/1.0',
eclipse: 'scroll-eclipsehelp/1.0',
epub: 'scroll-epub/1.0'
};
if (!!~Object.keys(that.restUrls).indexOf(that.exporter)) {
that.exportScheme = jQuery(that.link.target).data('export-scheme');
that.pageId = jQuery('[data-exporter]').data('page-id');
that.url = that.restUrl + '/' + that.restUrls[that.exporter] + '/export?exportSchemeId=' + that.exportScheme + '&rootPageId=' + that.pageId;
that.startExport();
}
};
this.poll = function () {
that.pollTimeout = setTimeout(function () {
jQuery.get(that.restUrl + '/' + that.restUrls[that.exporter] + '/async-tasks/' + that.job.id, function (response) {
that.updateDialog(response);
if (!response.finished && !that.finished) {
that.poll();
} else {
that.finishExport(response);
}
});
}, that.pollInterval);
};
this.startExport = function () {
that.openDialog();
jQuery(that.hash + ' #dialog-close-button').text('Cancel');
jQuery(that.hash + ' #dialog-close-button').click(function () {
AJS.dialog2(that.hash).hide();
});
jQuery.get(that.url, function (job) {
console.log(job);
that.job = job;
that.poll();
});
};
this.finishExport = function (response) {
that.finished = true;
var download = that.restUrl + '/' + that.restUrls[that.exporter] + '/export/' + that.job.id + '/' + response.filename;
jQuery(that.hash + ' .status-message').html('Export finished - <a class="aui-button aui-button-link" href="' + download + '">download</a>');
jQuery(that.hash + ' #dialog-close-button').text('Close');
window.location = download;
};
this.openDialog = function () {
AJS.dialog2(that.hash).show();
AJS.progressBars.setIndeterminate("#progress-bar");
var text = 'Exporting to ' + that.exporter.toUpperCase();
jQuery(that.hash + ' .aui-dialog2-header-main').text(text);
jQuery(that.hash + ' .status-message').html('Waiting for export to begin');
AJS.dialog2(that.hash).on('hide', function () {
if (!that.finished && that.job) {
that.finished = true;
clearTimeout(that.pollTimeout);
jQuery.ajax({
url: that.restUrl + '/' + that.restUrls[that.exporter] + '/async-tasks/' + that.job.id,
type: 'DELETE'
});
}
});
};
this.updateDialog = function (response) {
AJS.progressBars.update("#progress-bar", parseFloat(response.progress / 100));
jQuery(that.hash + ' .status-message').html(response.message);
};
this.init();
};
jQuery('[data-exporter]').on('click', function (link) {
new openExporter(link);
});
}
});
Usage
Any anchor element with the data attribute data-exporter
is targeted for exports.
Example
<a href="#demo-dialog" data-exporter="pdf" data-export-scheme="bundled_default" data-page-id="$page.id">
EXPORT TO PDF
</a>
The href
has to match the id
of the dialog provided for the export.
Data Attributes
Name | Value |
---|---|
| Selects the export scheme you want to use for exporting (including the template and all your adapted settings). Usually, Example: |
| Selects the Scroll Exporter to use for exporting. The following values are supported as long as the respective Scroll Exporter is installed: |
| Selects the root page of the export. The specified export scheme defines what pages actually will be exported. Typically you'll want to use the |